注意
前往結尾下載完整的範例程式碼。或透過 JupyterLite 或 Binder 在您的瀏覽器中執行此範例
單調約束#
此範例說明單調約束對梯度提升估計器的影響。
我們建立一個人工資料集,其中目標值通常與第一個特徵呈正相關(具有一些隨機和非隨機變化),並且通常與第二個特徵呈負相關。
透過在學習過程中分別對特徵施加單調增加或單調減少約束,估計器能夠正確遵循總體趨勢,而不是受變化的影響。
此範例的靈感來自 XGBoost 文件。
# Authors: The scikit-learn developers
# SPDX-License-Identifier: BSD-3-Clause
import matplotlib.pyplot as plt
import numpy as np
from sklearn.ensemble import HistGradientBoostingRegressor
from sklearn.inspection import PartialDependenceDisplay
rng = np.random.RandomState(0)
n_samples = 1000
f_0 = rng.rand(n_samples)
f_1 = rng.rand(n_samples)
X = np.c_[f_0, f_1]
noise = rng.normal(loc=0.0, scale=0.01, size=n_samples)
# y is positively correlated with f_0, and negatively correlated with f_1
y = 5 * f_0 + np.sin(10 * np.pi * f_0) - 5 * f_1 - np.cos(10 * np.pi * f_1) + noise
在此資料集上擬合第一個沒有任何約束的模型。
gbdt_no_cst = HistGradientBoostingRegressor()
gbdt_no_cst.fit(X, y)
在此資料集上擬合第二個模型,分別具有單調增加 (1) 和單調減少 (-1) 約束。
gbdt_with_monotonic_cst = HistGradientBoostingRegressor(monotonic_cst=[1, -1])
gbdt_with_monotonic_cst.fit(X, y)
讓我們顯示預測在兩個特徵上的部分依賴性。
fig, ax = plt.subplots()
disp = PartialDependenceDisplay.from_estimator(
gbdt_no_cst,
X,
features=[0, 1],
feature_names=(
"First feature",
"Second feature",
),
line_kw={"linewidth": 4, "label": "unconstrained", "color": "tab:blue"},
ax=ax,
)
PartialDependenceDisplay.from_estimator(
gbdt_with_monotonic_cst,
X,
features=[0, 1],
line_kw={"linewidth": 4, "label": "constrained", "color": "tab:orange"},
ax=disp.axes_,
)
for f_idx in (0, 1):
disp.axes_[0, f_idx].plot(
X[:, f_idx], y, "o", alpha=0.3, zorder=-1, color="tab:green"
)
disp.axes_[0, f_idx].set_ylim(-6, 6)
plt.legend()
fig.suptitle("Monotonic constraints effect on partial dependences")
plt.show()
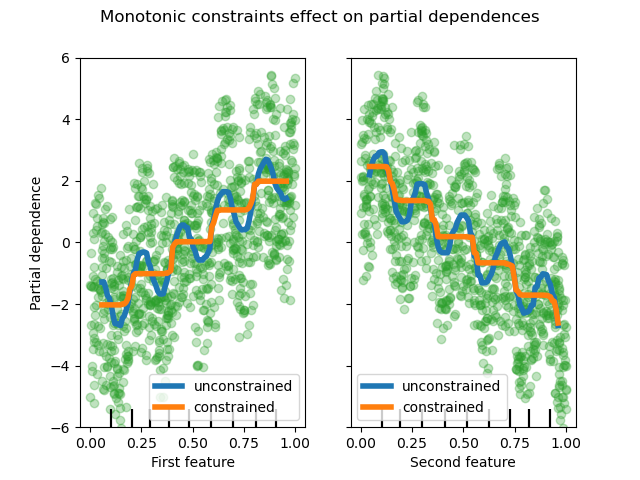
我們可以發現,未受約束模型的預測捕獲了資料的震盪,而受約束模型遵循總體趨勢並忽略局部變化。
使用特徵名稱指定單調約束#
請注意,如果訓練資料具有特徵名稱,則可以透過傳遞字典來指定單調約束
import pandas as pd
X_df = pd.DataFrame(X, columns=["f_0", "f_1"])
gbdt_with_monotonic_cst_df = HistGradientBoostingRegressor(
monotonic_cst={"f_0": 1, "f_1": -1}
).fit(X_df, y)
np.allclose(
gbdt_with_monotonic_cst_df.predict(X_df), gbdt_with_monotonic_cst.predict(X)
)
True
指令碼的總執行時間:(0 分鐘 0.587 秒)
相關範例